320x100
https://leetcode.com/problems/rotate-list/
Rotate List - LeetCode
Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview.
leetcode.com
node 목록과 임의의 정수가 주어졌을 때, 임의의 정수만큼 목록을 rotation 시켜 목록을 반환하는 문제다.
예상과 정답이 다르지 않은 방법이였다.
[C++]
/**
* Definition for singly-linked list.
* struct ListNode {
* int val;
* ListNode *next;
* ListNode() : val(0), next(nullptr) {}
* ListNode(int x) : val(x), next(nullptr) {}
* ListNode(int x, ListNode *next) : val(x), next(next) {}
* };
*/
class Solution {
public:
ListNode* solve(ListNode* node) {
ListNode* head = node;
while (head->next->next) {
head = head->next;
}
ListNode* tail = head->next;
head->next = nullptr;
tail->next = node;
return tail;
}
ListNode* rotateRight(ListNode* head, int k) {
if (!head || !head->next) {
return head;
}
ListNode* backup = head;
int len = 1;
while (head->next) {
++len; head = head->next;
}
head = backup;
for (int i=0; i<k%len; ++i) {
head = solve(head);
}
return head;
}
};
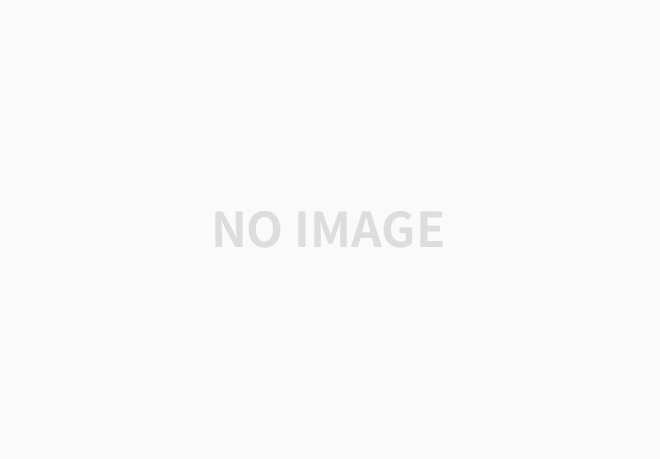
# Definition for singly-linked list.
# class ListNode:
# def __init__(self, val=0, next=None):
# self.val = val
# self.next = next
class Solution:
def rotateRight(self, head: Optional[ListNode], k: int) -> Optional[ListNode]:
if not head or not head.next:
return head
backup = head
length = 1
while head.next:
length += 1
head = head.next
# set infinite rotatin
head.next = backup
head = backup
k = k % length
# rotation 돌수록 가장 tail 쪽 노드부터 head가 되므로 length - k - 1
# rotate = 1
# 5 - 1 - 1 = 3
for _ in range(length - k - 1):
head = head.next
# anwer = head.next = node(4)
answer = head.next
# answer.next = node(3).next = None
head.next = None
return answer
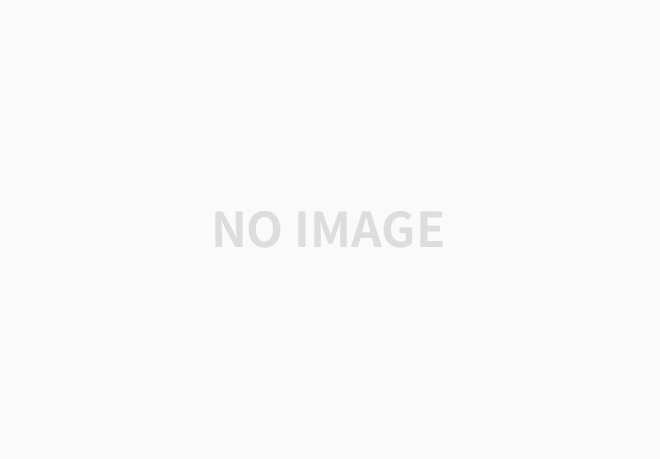
320x100
'코딩테스트 준비 > leetcode' 카테고리의 다른 글
75. Sort Colors (0) | 2021.08.22 |
---|---|
62. Unique Paths (0) | 2021.08.21 |
86. Partition List (0) | 2021.08.19 |
78. Subsets (0) | 2021.08.18 |
141. Linked List Cycle (0) | 2021.08.17 |